Spring Boot Reactive API Part 2
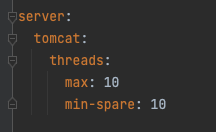
Spring Boot Reactive: Improving CPU bound performance using Scheduler In part 1 , we have seen that Spring Boot Reactive doesn't really improve performance if the WAITING time is CPU bound / CPU intensive tasks. This article show a quick way on how we can improve the speed through specific configuration Setup Spring Boot's embedded Tomcat is reconfigured to only use 10 worker threads, this way we can clearly see the performance limitation and tweaks. Gatling is configured to simulate 30 concurrent requests with 30000 milliseconds request timeout. APIs internal process is calling java native code that calculate the value of PI over 1 million iterations Default / No scheduler The main reason why CPU bound tasks do not improve (or even degrade) with high parallelism is because of the costly overhead for context switching in the low level. In the scenario below, 10 main threads are all being used to calculate PI values and because there are less CPU than threads, a lot of context